Intellisense
The developers (included the future you) that are going to use the custom command could be helped with some autosuggestions. How could we leverage VS Code Intellisense to document our custom command?
We need to use a TypeScript definition file to add them, you just need to:
create an
index.d.ts
file into thecypress/support/signup
directory (where thesignup-v1.js
file is)add the typing itself. We are not going to speak about TypeScript itself, just take a look at the result in terms of the file itself
File: cypress/support/signup/index.d.ts
/// <reference types="Cypress" />
declare namespace Cypress {
interface Chainable<Subject> {
/**
* Register a new user
* @example
* cy.signupv1()
* cy.signupv1({email: "foo[AT]bar.io", username: "Foo", password: "ar"})
* cy.signupv1().then(user => { ... })
*/
signupV1(user?: {
email?: string;
username?: string;
password?: string;
}): Chainable<{
email: string;
username: string;
password: string;
}>;
/**
* Register a new user or leverage the previously registered one (if possible)
* @example
* cy.signupv3()
* cy.signupv3({email: "foo[AT]bar.io", username: "Foo", password: "ar"})
* cy.signupv3().then(user => { ... })
* @example
* // the second call with the same (or empty) user data gets the user authenticated without
* // registering a new one
* cy.signupv3()
* cy.signupv3() // the user will not be registered twice
* cy.signupv3({ignoreLocalStorage: true}) // force the user be registered
* cy.signupv3({email: "foo[AT]bar.io", username: "Foo", password: "ar"})
* cy.signupv3({email: "foo[AT]bar.io", username: "Foo", password: "ar"}) // the user will not be registered twice
* cy.signupv3({email: "foo[AT]bar.io", username: "Foo", password: "ar"}, ignoreLocalStorage: true) // force the user be registered
*/
signupV3(user?: {
email?: string;
username?: string;
password?: string;
ignoreLocalStorage?: boolean = false;
}): Chainable<{
email: string;
username: string;
password: string;
}>;
}
}
and the hints that it gives you while coding your test:


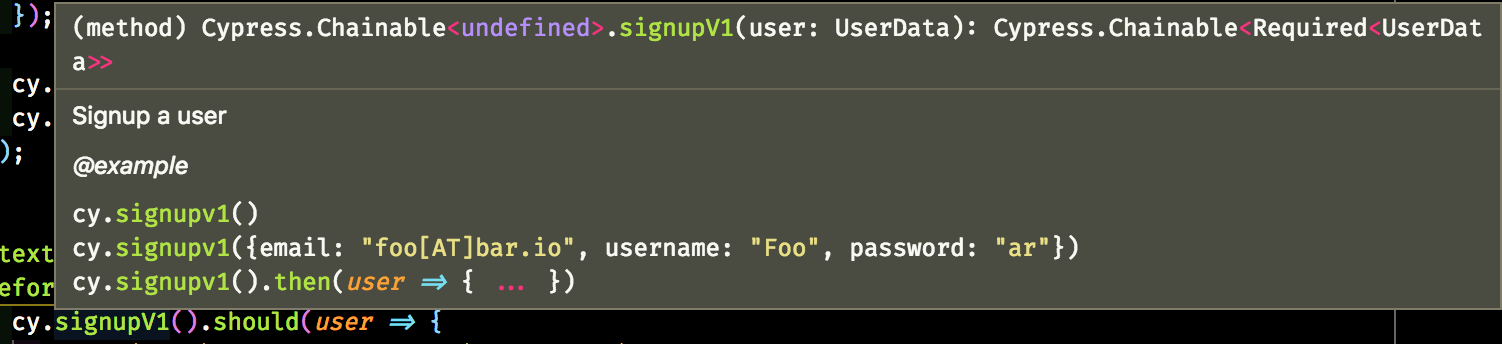
TypeScript can do more than documenting your function utilities, but for the sake of our goal just remember that:
- almost every Cypress command must be defined into the
namespace Cypress
block
declare namespace Cypress {
interface Chainable<Subject> {
// ...
}
}
- the question marks before the type definition make the variable optional (you are not forced to pass the user data to the custom command)
signupV1(user?: {
email?: string;
username?: string;
password?: string;
})
- the commands chained after the
cy.signupV1
command are going to receive the registered user details, that's why the return type of the function is
Chainable<{
email: string;
username: string;
password: string;
}>
Author: Stefano Magni